Step 3.3: SDK Setup
Initialising SDK
The Push SDK has to be initialised in the App Delegate class
In AppDelegate
add import PushSDK
Create two references to hold the SDK and PushologiesUserNotificationDelegate (
PushologiesCUserNotificationDelegate` for Obj-C).
var sdk: Pushologies?
var userNotificationDelegate: PushologiesUserNotificationDelegate?
Pushologies *sdk;
PushologiesCUserNotificationDelegate *sdkUserNotificationDelegate;
In the lifecycle method**didFinishLaunchingWithOptions**
instantiate the SDK, using the API credentials gathered in previous steps.
// Inject the credentials
sdk = Pushologies(
apiKey: “MyPushologiesAPIKey”,
apiSecret: “MyPushologiesSecretKey”,
inAppOpenDelay: 5000,
tenantID: “MyTenantID”
)
// Set the UserNotificaionDelegate
userNotificationDelegate = PushologiesUserNotificationDelegate(sdk: sdk)
UNUserNotificationCenter.current().delegate = userNotificationDelegate
// Set CustomerID (optional)
sdk?.customerID = "YourID"
sdk = [
Pushologies.alloc
initWithApiKey: infoDict[@"API_KEY"]
apiSecret: infoDict[@"API_SECRET_KEY"]
inAppOpenDelay: infoDict[@"IN_APP_OPEN_DELAY"]
tenantID: infoDict[@"TENANT_ID"]
];
sdkUserNotificationDelegate = [
AppUserDelegate.alloc
initWithSdk: sdk
];
UNUserNotificationCenter.currentNotificationCenter.delegate = sdkUserNotificationDelegate;
sdk.customerID = @"YourCustomIDHere";
Add authorisation prompts after initialising the SDK, this includes notification and location permission. This is optional and can be called at any place inside your app based on your convenience.
// Use whichever prompts your application requires
Task {
await sdk?.showPushNotificationAuthorisationPrompt()
}
Task {
await sdk?.showLocationAuthorisationPrompt()
}
Task {
// altertnatively use below to prompt both in one call
await sdk?.showAuthorisationPrompts()
}
[sdk showAuthorisationPromptsWithCompletionHandler:^{}];
Please use appropriate Purpose String for your location permission. Otherwise the prompts may not appear in your device.
To find your deviceID please use the below method
sdk?.deviceID
Print your deviceID in our console and check your device info in the portal.
Find your device in the subscriber explorer
Portal: https://portal.pushologies.com/subscriber-explorer
You should be able to see your device information and session details as well. If you have any issues seeing your subscriber in the portal, please check our troubleshooting guide.
AppDelegate Lifecycle
If not already present add the following three lifecycle methods and call the SDK’s corresponding methods. Here is a complete example.
func application(
_ application: UIApplication,
didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data
) {
sdk?.application(
application,
didRegisterForRemoteNotificationsWithDeviceToken: deviceToken
)
}
func application(
_ application: UIApplication,
didReceiveRemoteNotification userInfo: [AnyHashable : Any],
fetchCompletionHandler completionHandler: @escaping (UIBackgroundFetchResult) -> Void
) {
sdk?.application(
application,
didReceiveRemoteNotification: userInfo,
fetchCompletionHandler: completionHandler
)
}
func application(
_ application: UIApplication,
didFailToRegisterForRemoteNotificationsWithError error: Error
) {
sdk?.application(
application,
didFailToRegisterForRemoteNotificationsWithError: error
)
}
func application(
_ application: UIApplication,
handleEventsForBackgroundURLSession identifier: String,
completionHandler: @escaping () -> Void
) {
sdk?.application(
application,
handleEventsForBackgroundURLSession: identifier,
completionHandler: completionHandler
)
}
- (void)application:(UIApplication *)application didRegisterForRemoteNotificationsWithDeviceToken:(NSData *)deviceToken {
[sdk application:application didRegisterForRemoteNotificationsWithDeviceToken:deviceToken];
}
- (void)application:(UIApplication *)application didReceiveRemoteNotification:(NSDictionary *)userInfo fetchCompletionHandler:(void (^)(UIBackgroundFetchResult))completionHandler {
[sdk application:application didReceiveRemoteNotification:userInfo fetchCompletionHandler:completionHandler];
}
- (void)application:(UIApplication *)application didFailToRegisterForRemoteNotificationsWithError:(NSError *)error {
[sdk application:application didFailToRegisterForRemoteNotificationsWithError:error];
}
- (void)application:(UIApplication *)application handleEventsForBackgroundURLSession:(NSString *)identifier completionHandler:(void (^)(void))completionHandler {
[sdk application:application handleEventsForBackgroundURLSession:identifier completionHandler:completionHandler];
}
Notification Service Extension
Navigate to the NotificationService class
Replace the above NotificationService class with this new class that inherits from PSDKServiceExtension
.
import PushSDK
class NotificationServiceExtension: PSDKServiceExtension {
override func didReceive(
_ request: UNNotificationRequest,
withContentHandler contentHandler: @escaping (UNNotificationContent) -> Void
) {
if request.isPushologiesRequest {
super.didReceive(request, withContentHandler: contentHandler)
}
}
}
#import "NotificationService.h"
#import <PushSDK/PushSDK.h>
@interface NotificationService ()
@property (nonatomic, strong) void (^contentHandler)(UNNotificationContent *contentToDeliver);
@property (nonatomic, strong) UNMutableNotificationContent *bestAttemptContent;
@end
@implementation NotificationService
PSDKServiceExtension *serviceExtension;
- (instancetype)init
{
self = [super init];
if (self) {
serviceExtension = [PSDKServiceExtension.alloc init];
}
return self;
}
- (void)didReceiveNotificationRequest:(UNNotificationRequest *)request withContentHandler:(void (^)(UNNotificationContent * _Nonnull))contentHandler {
if (request.isPushologiesRequest) {
[serviceExtension didReceiveNotificationRequest:request withContentHandler:contentHandler];
}
}
- (void)serviceExtensionTimeWillExpire {
[serviceExtension serviceExtensionTimeWillExpire];
}
@end
Now the class will look like this
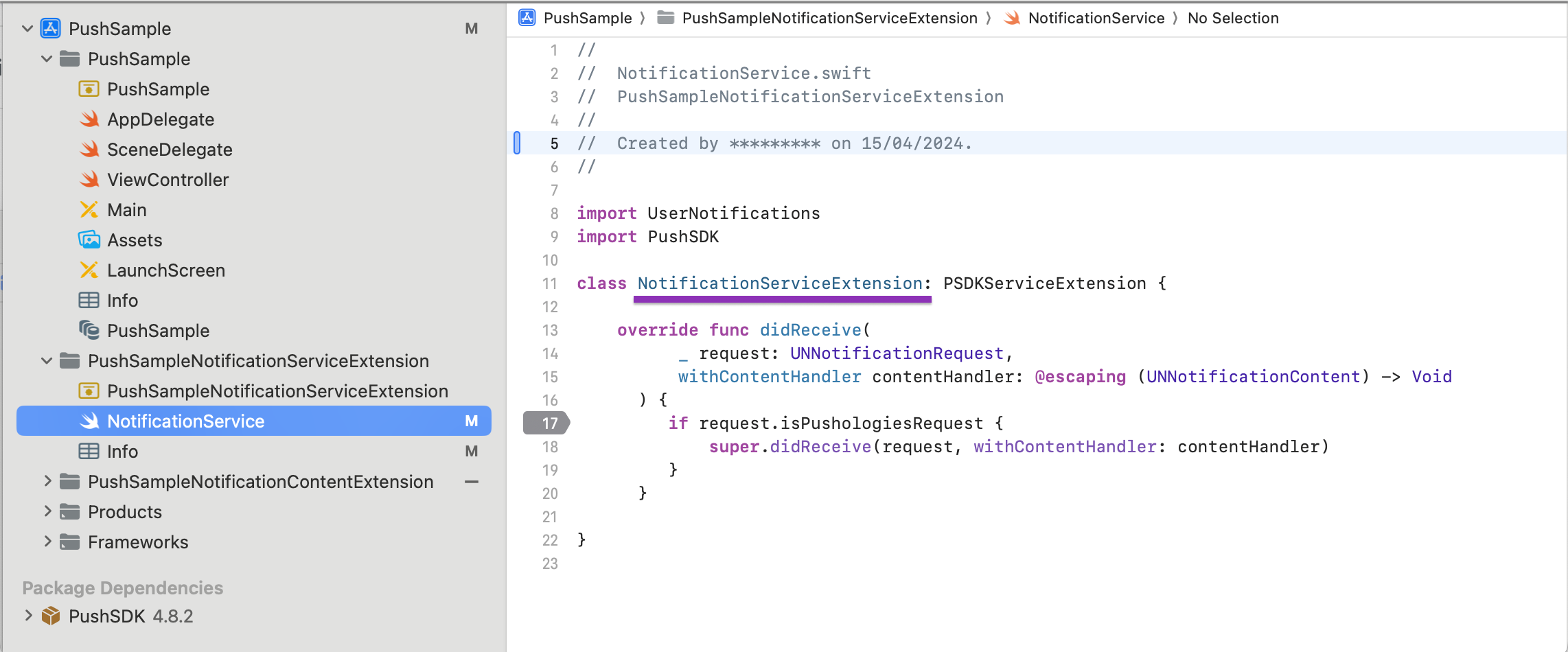
Include the PushologiesAppGroup and UNNotificationExtensionCategory into the NotificationServiceExtension's info.plist file
Add this string PSDKNotification under UNNotificationExtensionCategory Array
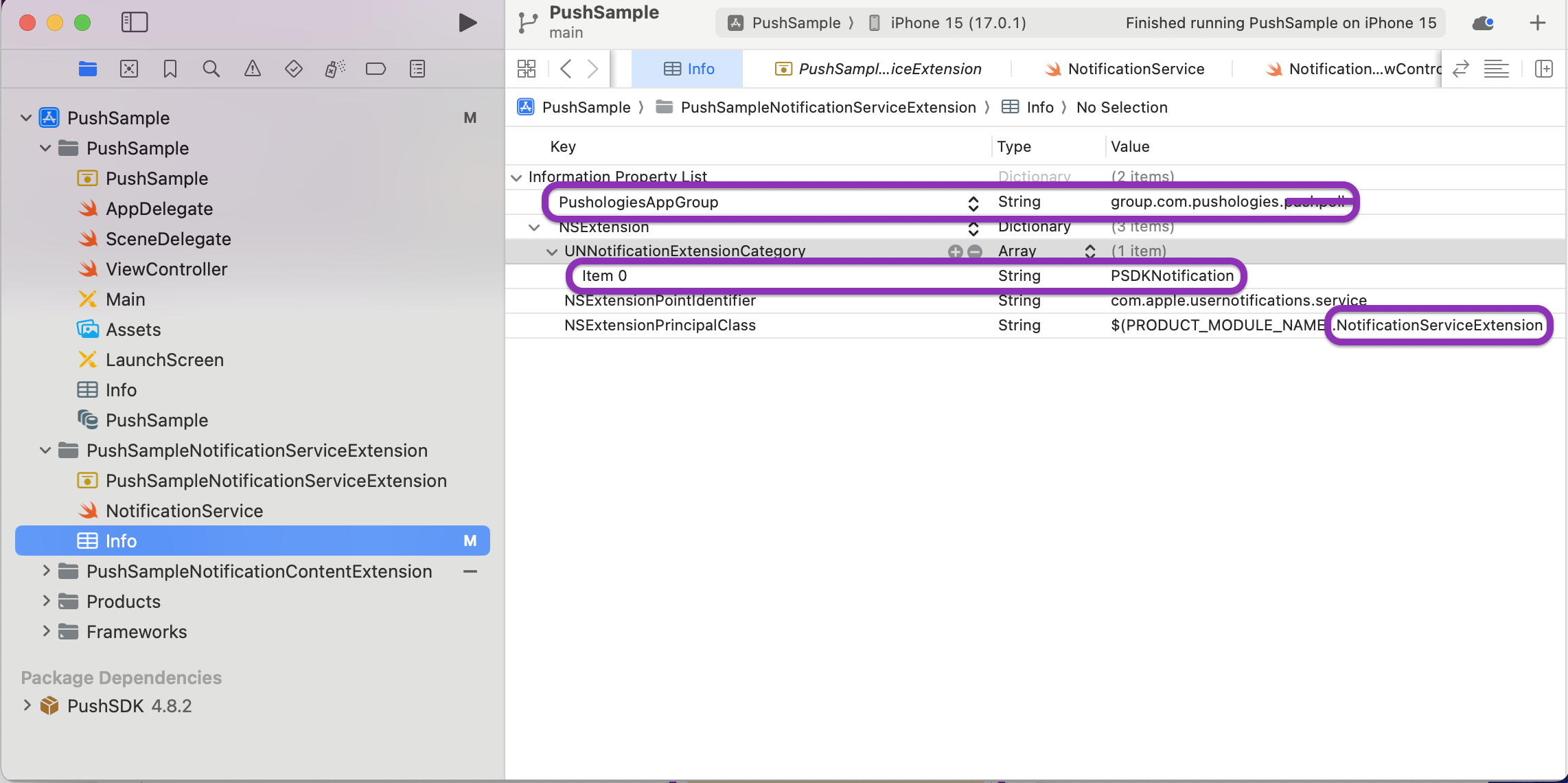
Also make sure the NSExtensionPrincipalClass has the same name of the NotificationServiceExtension class.
Test your first Push Notification
Its time to send a standard or a video push notification from the portal to the device. On successfully receiving your first notification please check its status in the subscriber event history tab.
Notification Content Extension
Navigate to the NotificationViewController and makes sure you remove all UI elements and the class should be left empty.
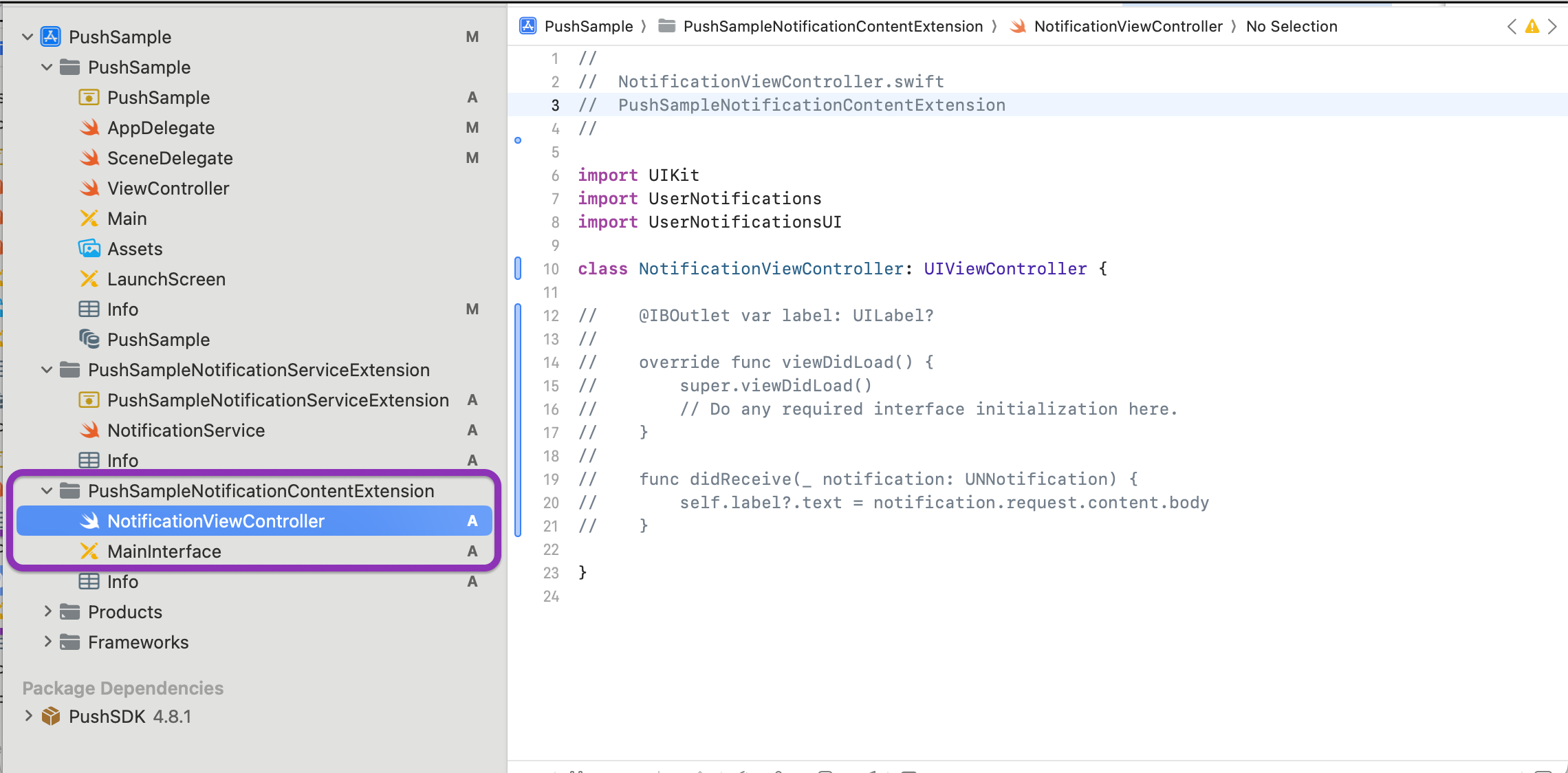
Now in MainInterface, please remove any UI elements found by default. For example, the default label shown below should be removed from the View. Now lets make the Notification View Controller inherit from Class CarouselNotificationContentViewController and Module PushSDK
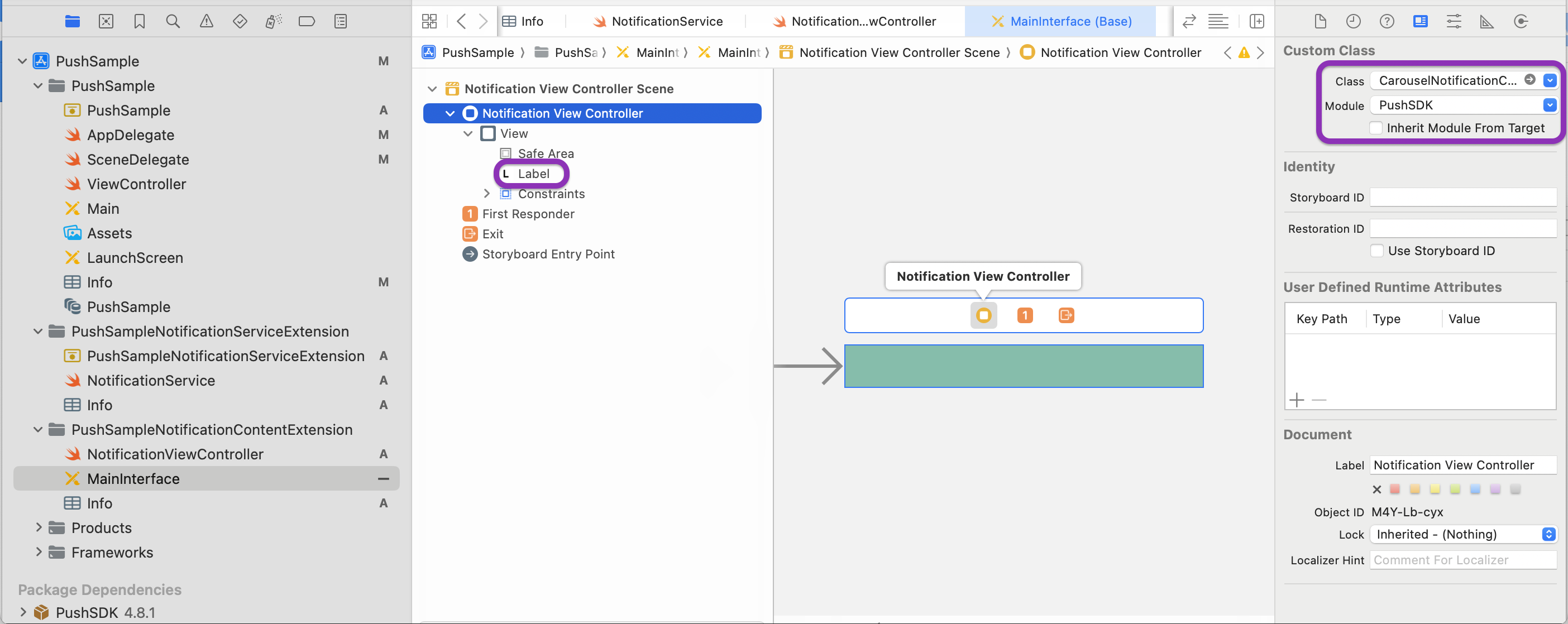
Include the PushologiesAppGroup into the NotificationContentExtension's info.plist file
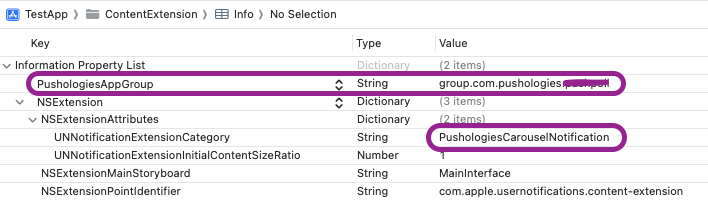
Test your Carousel Notifications now
You should be able to see Carousel notifications with preview.
Please see the troubleshooting guide if you have any issues
Updated 25 days ago